Get to know all about Unity’s Random Function including the use case, advantages, disadvantages, and a lot more.

If we just peek into the past of Random Numbers and the Evolution of Mathematics in the Computer world then we would find evidence of the first random number ever produced was in 1946 by John von Neumann. Since then, the process of getting a random number and the use case has evolved into a more promising and accurate model which is now used by most of the applications. Random Function is a method in which the system generates a set of numbers from the given range and then arbitrarily selects a particular number from the given set depending upon the conditions or the probability.
Uses of Random Function
Random Function, as the name suggests, it’s basically all about unpredictable results. The use case of Random numbers could be found in a wide variety of applications especially from the fields of Games, Gambling, Computer Simulation, Mathematical Modelling, Cryptography and so on. The random function helps the following application to excel with the logic and create instances of unpredictable results. In the field of Game Development, Random Function is a crucial element for Game Developers to add on the extra fun and dynamic element that makes the game feel alive. In Unity, Random Function is widely used for a variety of purposes like instantiating random spawn points, random behaviour of NPC, randomness in the gameplay, and so on. This makes sure that the game is not hardcoded to a single thing but is responsive enough to change over time so that it stays fresh even if we play hundreds of times. For example, in One Dash the spawn of coins was set to random instantiating depending upon a few set of rules. This ensures that the user playing the game will never get a coin every time on a particular point, rather the spawn rate and the spawn position would be randomly making it more fun to play.
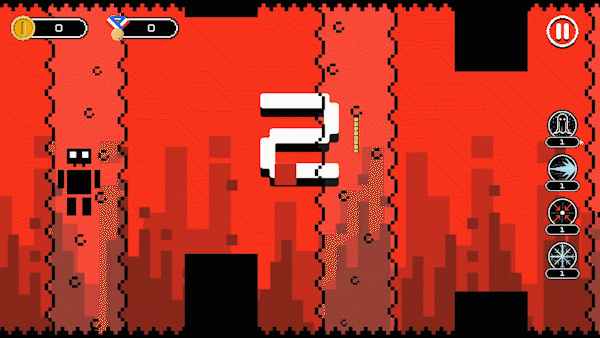
Check One Dash now.
But before we dive deep into this topic, it’s essential to know about the difference between the Unity Random Function and Microsoft Random Function. However, they might sound the same but the use case and the way of working are different which might be a problem for many Unity users if not chosen right.
UnityEngine.Random vs System.Random
Although Microsoft was the first to develop the Random Function, the game never ended there. As Unity being a Game Engine has a separate Random Function, it surely has some bigger hand over the default System.Random. This creates a separation layer between the way of using both the Function and hence leave us in a dilemma of choosing the right one for our game. But this is, however, not a great deal to tackle if we get to know how these two Dedicated Functions actually work.
UnityEngine.Random
Unity uses the static class for all the calls to Random Function making it easier for the user to use it anywhere in the game without the tension of instantiating a game object. That simply means, calling a single line of Random function logic would basically do all the work. Unity has some pre-made and ready to use functions ranging from getting Random numbers from a given range to getting Random points inside a Sphere. Unity got us covered with the easiest solution that we no need to worry about. But if everything is fine about Unity’s Random Function then how far the System.Range is good for us?
System.Range
System.Range is the default C# code snippet that basically works with the help of instantiating an object which would be further used for generating and storing the Random Numbers. As we are creating the instances through the objects, we get at a free hand on creating more than one instance of random numbers. This also means that it’s easier to create a reproducible stream of a random number from a given seed. Due to such advantages over the UnityEngine.Random, System.Range is comparably more advanced and has more control.
So now we got a clear picture that both the Unity Random Function and System Random function has it’s own working principle and it’s not the same for every condition to use them without proper knowledge. Do let us know if you want a more in-depth blog on this topic, we would love to write one!
Now that being said let check all the possible ways how we can use the Random Function of Unity for our Games.
Static Properties
insideUnitCircle - This returns a Random Point in a circle having a Unit Radius.
insideUnitSphere – This returns a Random Point inside a Sphere having a Unit radius.
onUnitSphere - This returns a random point on the surface of the Sphere having a unit radius.
rotation – This return a Random Rotation.
rotationUniform – This returns a Random Rotation having a Uniform Distribution.
state – Gets/Sets the complete Internal state of the Random Number Generator.
value – This returns a random number between 0.0[Inclusive] and 1.0 [inclusive]. However, this is a read-only parameter.
Static Methods
ColorHSV – This function generates a random colour from the HSV and the alpha ranges.
InitState – This initializes the state of the random number with a given seed.
Range – This function returns the Random float number min [inclusive] and max [inclusive]. This is also a read-only parameter.
Uses of Random Function
Random Integer
If we want to get a Random number from a range of integers then the general syntax is as follows –
int randint = Random.Range(min,max);
Where int is the datatype, randint is the variable to store the data or information, Random.Range() is the function to get the random number from the static class by passing two arguments with datatype as an integer, min is the minimum integer to start which is inclusive and max is the maximum range of the integer which is exclusive. For the Random number generation as an integer, the minimum range is always inclusive which means it is considered and included by Unity for the condition and logic of the Random number generation whereas, the maximum range is always exclusive, which means it is excluded and the range taken by Unity is the max – 1. Given below is a small example to generate a random number from the given integer range.
int randint = Random.Range(1,11);
Here, as you can see the Range is given to be 1 as minimum and 11 as maximum, whereas Unity will return any random value between 1 and 10, this is because 11 is considered to be the exclusive limit.
Random Float
For the case of Floats, the Random Range function behaves as both inclusive limits. That means, both the minimum and maximum range are inclusive and any value we put in the range is considered by Unity for the Random number generation.
float randfloat = Random.Range(1f,10f);
The above line will return a random floating number between the range of 0f to 10f.
Random Position in Vector2
We can get an arbitrary point anywhere in the Vector2 coordinate using the Random Function. But we need to take care of the bounds so that the point is never created outside the camera.
Vector2 randpoint = new Vector2(Random.Range(1f, 5f), Random.Range(1f, 5f));
The above line creates a random point by first choosing from the Random Range in x coordinate and then choosing a point from the Y coordinate. After it gets a random value in x and y coordinate the point is created in the Vecto2 space. This can help 2D games to instantiate objects on random points to add more complexity while making the game more dynamic.
Random Position in Vector3
The same above method can be applied for creating Random position on Vector3 Space too.
Vector3 randpoint = new Vector3(Random.Range(1f, 5f), Random.Range(1f, 5f), Random.Range(1f, 5f));
As we can see by adding the third coordinate which is the z-coordinate, we potentially changed the above line to work with Random point on Vector3 space.
Random Function for Arrays
Random function for the Arrays is very crucial and handy to use in most of the applications where they require to select particular data from a given set of data stored in an Array. Just like the above example, it’s not that hard but a bit tricky to understand and use it correctly.
First, we need to clear about the indexing of the Arrays. When it is said that an Array has 10 elements, Array[10] then the Array goes from element 0 to 9 instead of element 1 to 10 i.e, Array[0] = First element and Array[9] is the 10th data of the Array. And as Array elements are of integer it’s necessary for the Random Range to have the limit correctly or else we might encounter errors. Here is a small example of how you can use Random function for the Arrays.
int[] array;
var element = array[Random.Range(0, array.Length)];
From the above code snippet, we can see that the element has a datatype of variable which will be responsible to store the information about the data derived from the Array element. We are using the Random Function inside the Array starting from the minimum 0 as it is the first element and going to the array.Length which is the last element of the array. One thing to notice that we are not using the array.Length – 1 for the Random function even though it is for integer because as discussed above the Array element starts from 0 and it goes to total element – 1, which is what we require for the Random Function for integers.
Random point in a circle
With the help of Unity’s Static Methods, we can get directly a point inside a circle.
transform.position = Random.insideUnitCircle * radius;
where the radius is the radius of the circle and the Random.insideUnitCircle is the static property of the Unity Random function to get a random point inside a circle of the given radius taking 0 as the centre.
Random point in a Sphere
We can also get the Random point inside a sphere using Unity’s Static Methods available. For example –
transform.position = Random.insideUnitSphere * radius;
This line generates a random point inside a sphere of the given radius with a centre at 0.
Random point on a Sphere
We looked at how to create a random point inside a sphere but now what if we are required to create a random point on a sphere surface. Here is the way to create a random point on the surface of a sphere –
var point = Random.onUnitSphere * radius;
This line will return a Vecor3 position randomly from the surface of the sphere having a given radius.
Random Function for choosing Functions from an Array
Choosing randomly a function from a set of function to execute could be handy for games possessing a set of behaviours to trigger randomly. This could be easily done by storing all the desired function in an array and then creating a random number to decide which function to call in.
var functions = [func1, func2, func3];
functions[Random.Range(0, functions.Length)]();
This will trigger random function having their own set of logic and behaviour whenever required.
Random Color
We can get the Random Color by using the Static methods from the UnityRandom function easily. For example –
GetComponent<SpriteRenderer>().color = Random.ColorHSV(hueMin, hueMax, saturationMin, saturationMax, valueMin, valueMax);
Or,
GetComponent<Renderer>().material.color = Random.ColorHSV(hueMin, hueMax, saturationMin, saturationMax, valueMin, valueMax);
Where,
hueMin Minimum hue [0..1].
hueMax Maximum hue [0..1].
saturationMin Minimum saturation [0..1].
saturationMax Maximum saturation[0..1].
valueMin Minimum value [0..1].
valueMax Maximum value [0..1].
alphaMin Minimum alpha [0..1].
alphaMax Maximum alpha [0..1].
This method hence creates a random colour from the given HSV range and the alpha range.
Random Value between 0 and 1
Getting random values between a default range of 0 and 1 is easy using the Static Method Random.value.
var x = Random.value;
It returns a floating number anywhere between the 0 and 1;
Random number for different probability
Getting an unpredictable number depending upon the probability has been always an important logic for games to introduce variation in the gameplay. Normally Unity’s Random function generates number arbitrarily from a given range without any condition of probability which lacks control. So, here is an easy way how you can get the values 0 and 1 depending upon the probability ranges you have specified.
float[] probability = { 10f, 90f};
float Choose(float[] probs)
{
float total = 0;
foreach (float elem in probs)
{
total += elem;
}
float randomPoint = Random.value * total;
for (int i = 0; i < probs.Length; i++)
{
if (randomPoint < probs[i])
{
return i;
}
else
{
randomPoint -= probs[i];
}
}
return probs.Length - 1;
}
You can call the above function by providing the probability of the given number and could get the result accordingly.
Debug.Log(Choose(probability).ToString());
Here you will notice that value 1 prints most of the time as the probability of the value 1 is 90% and the probability of the value 0 printed is only 10%. Likewise, as you go on increasing the probability ranges, the values will change depending upon the probability specified.
Random shuffling a list
Shuffling word as we all know is mostly used in the game of cards. And this is, however, the core logic for the cards game, how well they can do it. So let’s see how we can do a random shuffle of a deck and get the result.
int[] deck = { 10, 20, 30 };
void Shuffle(int[] deck)
{
for (int i = 0; i < deck.Length; i++)
{
int temp = deck[i];
int randomIndex = Random.Range(0, deck.Length);
deck[i] = deck[randomIndex];
deck[randomIndex] = temp;
Debug.Log(deck[randomIndex]);
}
}
The above code snippet will give you the result of random shuffling of the deck every time you call the function. We have used a deck of integers for the ease of visualising the results, you can use anything you want to shuffle but don’t forget to first keep something in the deck!
Random picking numbers without repetition
Picking out Random numbers without repeating is an important concept of Random Functions. Most of the Games use it to pick a loot from the set of all the loot element and make sure to never repeat it again if the user has already claimed it. There are a lot of many use cases to this making it a crucial element in the Random number generation topic. So let’s check how we can do it easily.
List<int> list = new List<int>();
private void Start()
{
for (int n = 0; n < 10; n++)
{
list.Add(n);
}
}
Now after creating a list and populating with the numbers from 0 to 9 we will do some small calculation to get the random number from the list without any repetition.
void ChooseRandomNumber()
{
int index = Random.Range(0, list.Count - 1);
int i = list[index];
list.RemoveAt(index);
Debug.Log(i);
}
If we debug the above code then we will observe that we are getting random numbers from the list without any repetition. You can use the above method as many time you want until the list is empty. When there is no number left in the list to print, it will throw an argument overpass error which can be simply fixed by checking and generating the random number only when the list is not equal to zero.
For more information about the Random Functions Check Unity Official Page.
So far, we saw how the advancement in the field of Random Numbers has taken shape and how it has evolved as a crucial gameplay element. We have covered nearly everything related to Random function including the differences between UnityEngine.Random and System.Random, uses of Random Function in Game dev & other applications, and lastly How we can use Random Function for various purpose in Unity. Hope, this brief guide helps you ahead while working with Unity Random Function for your games.
Here are some essential resources to boost up your Game Development -
Get 100% Free Assets for your Games.
Get Started with your First Platformer Game.
Know more about Unity & more.
With Love From VOiD1 Gaming

Comments